Using the Shopee API can be challenging, especially when it comes to creating the sign
for authentication. This guide will help you generate the sign
with Python, retrieve the access_token
, and automate the process with n8n.
Step 1: Create an Account and App on Shopee Open Platform
- Go to Shopee Open Platform.
- Create an account and then create an app.
- Register your app for publishing and make sure it is approved.
Step 2: Get Shopee Code (Authorization Code)
The Shopee code is a one-time code used to retrieve the access_token
after the seller grants permission to access their shop. You can get the code through two methods: from the Shopee Open Platform Console or via API (/api/v2/shop/auth_partner
).
In this guide, we will focus on the first method for simplicity. Once you understand how to use the sign
and access_token
, you can switch to the second method if needed.
Steps to Get Shopee Code:
- Go to the App List on Shopee’s developer portal.
- Select Authorize for the app.
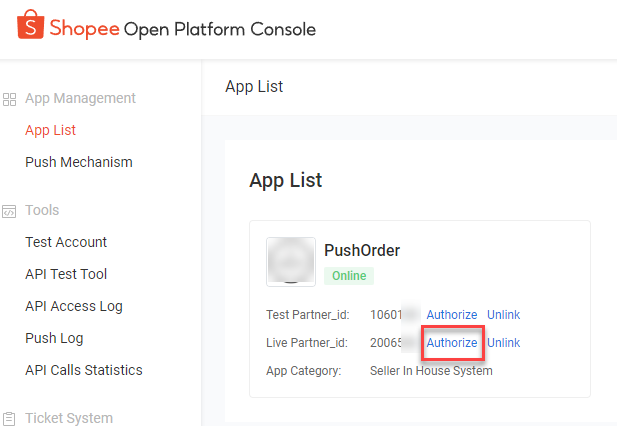
- Enter the homepage URL of the shop you need to authenticate.
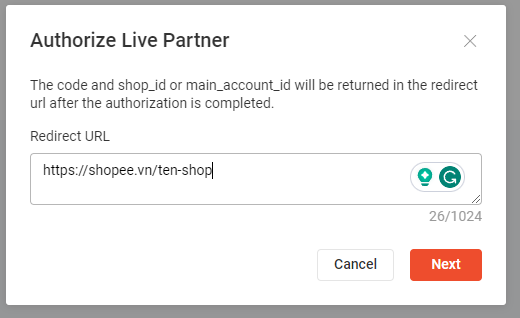
- Log in to the shop and follow the steps to grant permission.
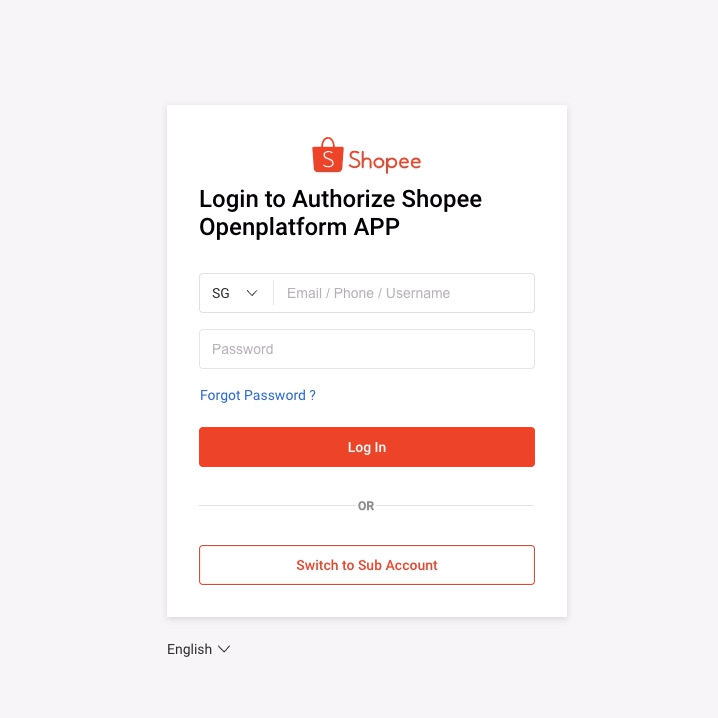
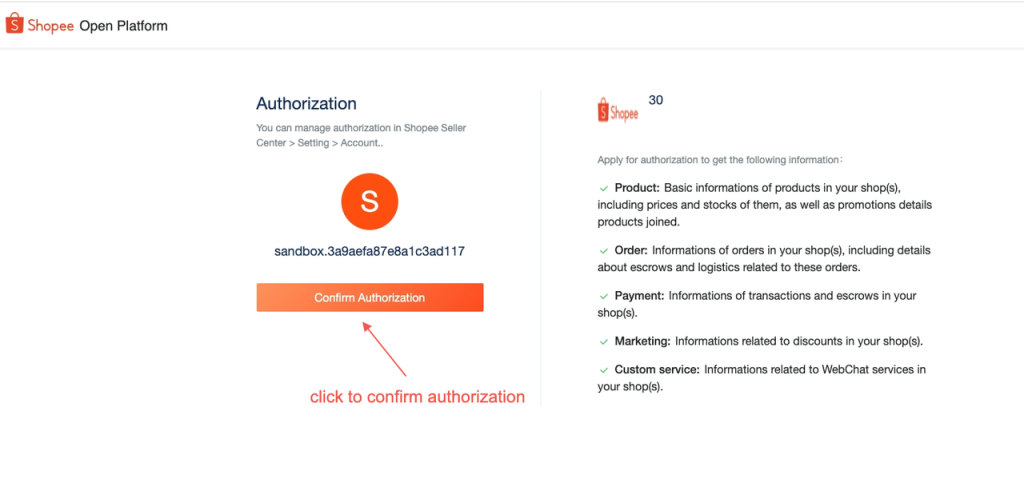
- After successful authorization, you will be redirected to a URL like this:rubyCopy code
https://open.shopee.com/?code=xxxxxxxxxx&shop_id=xxxxxx
- Copy the
code
from the URL and paste it into your Python script.
Step 3: Retrieve access_token
and refresh_token
Once you have the code
, you can use it to obtain the access_token
and refresh_token
.
Here’s the Python script to do that:
Python Script to Retrieve Tokens:
import time
import requests
import hmac
import hashlib
# Inputs
partner_id = "your_partner_id"
partner_key = "your_partner_key"
code = "authorization_code" # Replace with the code from the URL
shop_id = "your_shop_id"
path = "/api/v2/auth/token/get"
timestamp = int(time.time())
# Generate the sign
base_string = f"{partner_id}{path}{timestamp}"
sign = hmac.new(
partner_key.encode('utf-8'),
base_string.encode('utf-8'),
hashlib.sha256
).hexdigest()
# API Request to get tokens
url = f"https://partner.shopeemobile.com{path}"
payload = {
"partner_id": partner_id,
"code": code,
"shop_id": shop_id,
"sign": sign,
"timestamp": timestamp
}
response = requests.post(url, json=payload)
tokens = response.json()
# Access Token and Refresh Token
access_token = tokens.get("access_token")
refresh_token = tokens.get("refresh_token")
print(f"Access Token: {access_token}")
print(f"Refresh Token: {refresh_token}")
Note:
access_token
is valid for 4 hours.refresh_token
is valid for 1 month.
Step 4: Refresh the Access Token Automatically with n8n
When the access_token
expires, you can use n8n to refresh it automatically. Here’s how you can set up the workflow:
- Download and Import the n8n Workflow:
- Import the workflow file to your n8n instance.
- Configure the Workflow:
- Enter the required parameters (partner_id, partner_key, etc.).
- The workflow will request a new
access_token
andrefresh_token
and store them in a Lark table.
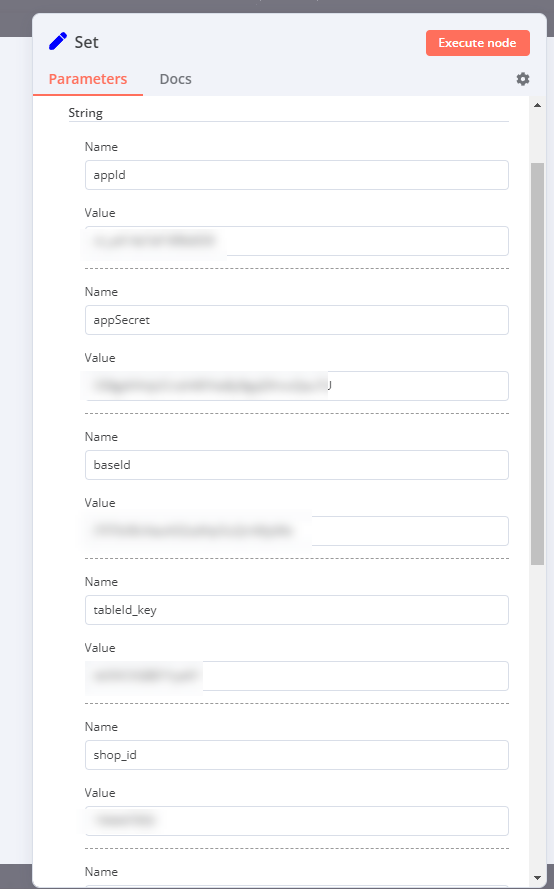
Step 5: Setting up the Lark Table
Create a table in Lark with the following columns:
access_token
refresh_token
You can retrieve the baseId
and tableId
from the Lark link, and use them to store the new tokens every time the API is called.

Step 6: Using the Access Token for API Calls
Once you have the access_token
, you can use it to call Shopee’s API. Every time you make an API request, retrieve the access_token
from the Lark table and use it in your API request headers.

Important Notes:
- Access Token Expiry: The
access_token
expires after 4 hours. You must refresh it periodically using therefresh_token
. - Refresh Token Expiry: The
refresh_token
expires after 1 month. If it expires, you’ll need to reauthorize the app to get a newcode
and repeat the process. - Security: Be cautious with storing your
partner_key
,access_token
, andrefresh_token
. Make sure they are not exposed in public repositories.
By following these steps, you can automate Shopee API authentication and manage tokens with ease using n8n.
n8n registration: here